THEORY :
"The Towers of Hanoi" is a mathematical puzzle which follows three basic rules :
1. Only one disk could be moved at a time.
2. A larger disk must never be stacked above a smaller one.
3. One and only one auxiliary needle could be used for the intermediate storage of disks.
Now, look at this figure ........
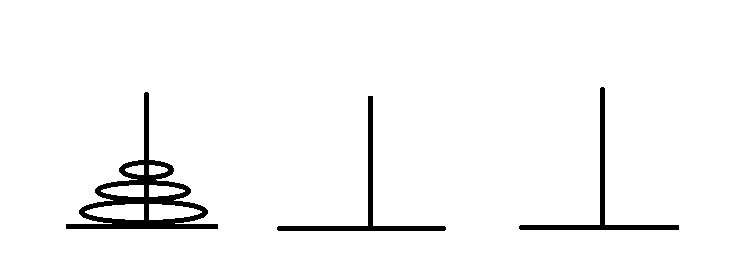
Source Auxiliary Destination
A B C
NOTE : Take your notebook and pen to understand the description below.
Imagine that we have to move only one disk , in this situation
we'll "move one disk form source(tower A) to destination(tower C).
When your first disk is moved , imagine that we have to move two disks.First, the top disk
is moved to auxiliary needle(tower B).Then the second disk is moved to the destination(tower C).
In this situation, your steps will be :
1."move one disk to auxiliary needle(tower B)"
2."move one disk to destination needle(tower C)"
3."move one disk from auxiliary(tower B) to destination needle(tower C)"
Now, to study the case for three disks......
follow these steps :
1."move two disks from source(tower A) to auxiliary needle(tower B)"
2."move one disk from source(tower A) to destination needle(tower C)"
3."move two disks from auxiliary(tower B) to destination needle(tower C)"
So,the generalized solution for this mathematical puzzle will be ..... .
STEP 1."move (N-1) disks from sourcce(tower A) to auxiliary needle(tower B)"
STEP 2."move one disk from source(tower A) to destination needle(tower C)"
STEP 3."move (N-1) disks from auxiliary(tower B) to destination needle(tower C)"
SOURCE CODE :
#include<stdio.h>
#include<conio.h>
void towerofhanoi(int n,char source,char des,char auxiliary)
{
//local declarations
static int step = 0;
//statements
printf("Towers (%d, %c, %c, %c)\n",n,source,dest,auxiliary);
if(n==1)
{
printf("\t\t\tStep %3d: Move from %c to %c\n",++step,source,dest);
}
else
{
towerofhanoi(n-1,source,auxiliary,dest); //General case
printf("\t\t\tStep %3d: Move from %c to %c\n",++step,source,dest); //Base case
towerofhanoi(n-1,source,auxiliary,dest); //General case
}
return;
getch();
}
************ THE END ***********
"The Towers of Hanoi" is a mathematical puzzle which follows three basic rules :
1. Only one disk could be moved at a time.
2. A larger disk must never be stacked above a smaller one.
3. One and only one auxiliary needle could be used for the intermediate storage of disks.
Now, look at this figure ........
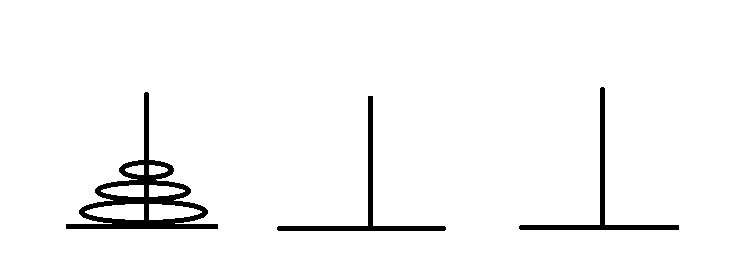
Source Auxiliary Destination
A B C
NOTE : Take your notebook and pen to understand the description below.
Imagine that we have to move only one disk , in this situation
we'll "move one disk form source(tower A) to destination(tower C).
When your first disk is moved , imagine that we have to move two disks.First, the top disk
is moved to auxiliary needle(tower B).Then the second disk is moved to the destination(tower C).
In this situation, your steps will be :
1."move one disk to auxiliary needle(tower B)"
2."move one disk to destination needle(tower C)"
3."move one disk from auxiliary(tower B) to destination needle(tower C)"
Now, to study the case for three disks......
follow these steps :
1."move two disks from source(tower A) to auxiliary needle(tower B)"
2."move one disk from source(tower A) to destination needle(tower C)"
3."move two disks from auxiliary(tower B) to destination needle(tower C)"
So,the generalized solution for this mathematical puzzle will be ..... .
STEP 1."move (N-1) disks from sourcce(tower A) to auxiliary needle(tower B)"
STEP 2."move one disk from source(tower A) to destination needle(tower C)"
STEP 3."move (N-1) disks from auxiliary(tower B) to destination needle(tower C)"
SOURCE CODE :
#include<stdio.h>
#include<conio.h>
void towerofhanoi(int n,char source,char des,char auxiliary)
{
//local declarations
static int step = 0;
//statements
printf("Towers (%d, %c, %c, %c)\n",n,source,dest,auxiliary);
if(n==1)
{
printf("\t\t\tStep %3d: Move from %c to %c\n",++step,source,dest);
}
else
{
towerofhanoi(n-1,source,auxiliary,dest); //General case
printf("\t\t\tStep %3d: Move from %c to %c\n",++step,source,dest); //Base case
towerofhanoi(n-1,source,auxiliary,dest); //General case
}
return;
getch();
}
************ THE END ***********